Bulk configure app details
Bulk configuration of app meta during on-boarding
While implementing Torii, you can bulk load app data you're currently managing in spreadsheets, such as IT app owners and contract owners, rather than manually inputting this information within each and every app.
This guide documents a Google Sheets example of how to bulk upload your application data into Torii using our APIs and embedded Apps Script code.
Below you will find:
- A live demo of syncing app owners into Torii
- A step-by-step configuration guide
Step 1 - Make a copy of the Google Sheet Example and make changes as required.
- Make a copy of the Bulk Update App Owners - EXAMPLE sheet.
Tip:
The Google Sheet examples all have read only permissions and should be copied into your own account.
- Select the Extensions menu item and then the Apps Script dropdown menu option.
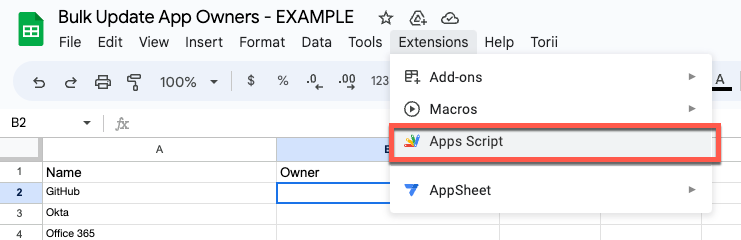
- Detailed documentation of the code can be found in the Bulk Update App Owners via API recipe below, followed by the Apps Script code.
Tip
The below App Script code can be modified as needed to support your requirements.
let API_KEY;
function onOpen() {
let ui = SpreadsheetApp.getUi();
ui.createMenu("Torii")
.addItem("Sync now", "syncNow")
.addItem("Reconfigure", "readConfig")
.addToUi();
}
function syncNow() {
let docProperties = PropertiesService.getDocumentProperties();
API_KEY = docProperties.getProperty("api_key");
if (!API_KEY) {
readConfig();
docProperties = PropertiesService.getDocumentProperties();
API_KEY = docProperties.getProperty("api_key");
}
syncAppOwners();
}
function readConfig() {
let ui = SpreadsheetApp.getUi();
let result = ui.prompt("Please enter Torii API Key");
API_KEY = result.getResponseText();
let docProperties = PropertiesService.getDocumentProperties();
docProperties.setProperty("api_key", API_KEY);
}
function syncAppOwners() {
let = sheet = SpreadsheetApp.getActive();
let data = sheet.getDataRange().getValues();
Logger.log(SpreadsheetApp.getActiveSheet().getName());
Logger.log(data);
data.shift();
let getAppsInOrg = getAllAppsInOrg();
data.forEach(function (col) {
let application = col[0];
let email = col[1];
let appCount = null;
let userCount = null;
let jsonResponse = JSON.parse(searchApps(application));
appCount = Object.keys(jsonResponse.apps).length;
console.log("AppCount " + appCount);
let idApp = null;
let idUser = null;
if (appCount >= 2) {
//Use Exact Name Match to Get AppID
let jsonResponse = JSON.parse(listApps(application));
Logger.log(jsonResponse);
idApp = jsonResponse.apps[0].id;
console.log(idApp);
} else if (appCount == 1) {
idApp = jsonResponse.apps[0].id;
console.log(idApp);
} else {
console.log("App " + application + " not found");
}
//Get the ID of the user
if (idApp) {
//Verify App is in Org before we proceed
if (getAppsInOrg.indexOf(idApp) != -1) {
console.log("Application is in Org " + idApp);
//GET USER ID
//First Check if the email is blank
if (email){
console.log ("email: " + email);
let jsonResponse = JSON.parse(getUser(email));
Logger.log(jsonResponse);
userCount = Object.keys(jsonResponse.users).length;
if (userCount == 1) {
idUser = jsonResponse.users[0].id;
console.log(idUser);
} else {
console.log("User " + email + " not found");
}
}
updateAppWithOwner(idApp, idUser);
} //EndidApp
} else {
console.log("Application is Not in Org " + idApp);
}
});
}
function searchApps(App_Name) {
let url = "https://api.toriihq.com/v1.0/apps/search?q=" + App_Name;
let response = UrlFetchApp.fetch(url, {
muteHttpExceptions: false,
method: "get",
contentType: "application/json",
headers: { Authorization: `Bearer ${API_KEY}` },
});
Logger.log(response);
return response;
}
function listApps(App_Name) {
let url = "https://api.toriihq.com/v1.0/apps?fields=id&name=" + App_Name;
let response = UrlFetchApp.fetch(url, {
muteHttpExceptions: false,
method: "get",
contentType: "application/json",
headers: { Authorization: `Bearer ${API_KEY}` },
});
Logger.log(response);
return response;
}
function getAllAppsInOrg() {
let allApps = [];
let url = "https://api.toriihq.com/v1.0/apps?fields=id";
console.log("Start get Apps");
let response = UrlFetchApp.fetch(url, {
muteHttpExceptions: false,
method: "get",
contentType: "application/json",
headers: { Authorization: `Bearer ${API_KEY}` },
});
let jsonResponse = JSON.parse(response);
for (var i = 0; i < Object.keys(jsonResponse.apps).length; i++) {
allApps.push(jsonResponse.apps[i].id);
}
return allApps;
}
function getUser(Email) {
let url = "https://api.toriihq.com/v1.0/users?email=" + Email;
let response = UrlFetchApp.fetch(url, {
muteHttpExceptions: false,
method: "get",
contentType: "application/json",
headers: { Authorization: `Bearer ${API_KEY}` },
});
Logger.log(response);
return response;
}
function updateAppWithOwner(App_ID, User_ID) {
let url = "https://api.toriihq.com/v1.0/apps/" + App_ID;
response = UrlFetchApp.fetch(url, {
muteHttpExceptions: false,
method: "put",
contentType: "application/json",
payload: JSON.stringify({
owner: User_ID,
}),
headers: {
Authorization: `Bearer ${API_KEY}`,
},
});
Logger.log(response);
}
- Update your Google Sheet to reflect the two required fields below:
Update the Name column with the application names you'd like to important the information from.
Then, update the Owner column with the email address of the application owner or IT owner.
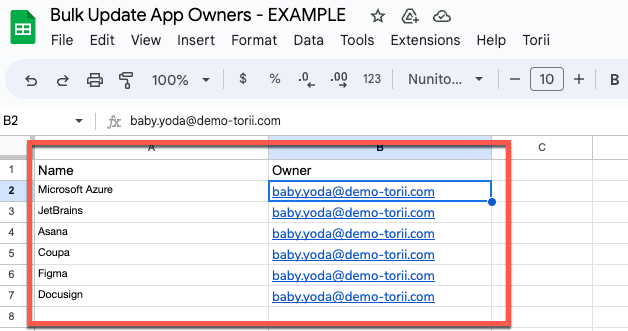
Tips
If additional fields are required they should be added and the code should be refactored based on the additional fields.
The recipe referenced in the previous step will provide detailed documentation of the code to help you make changes to suit your requirements.
Step 2 - Sync the data from the Google Sheet to Torii
- Select Torii from the lop line menu item and then Sync Now to run the code and push the data into Torii using the API.
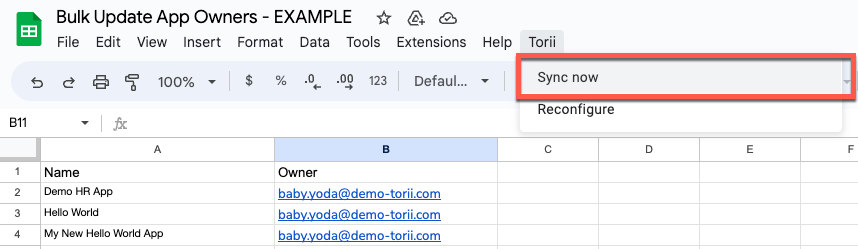
The first time you run Sync Now the embedded Apps Script will request permission for the code to run.
Authorization should be granted as shown below. You will need to re-select Sync Now once authorization has been granted.
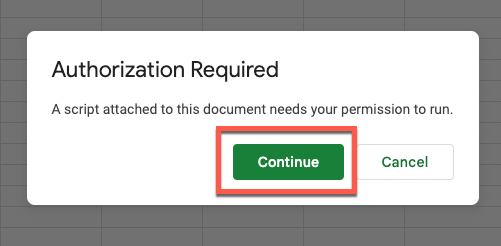
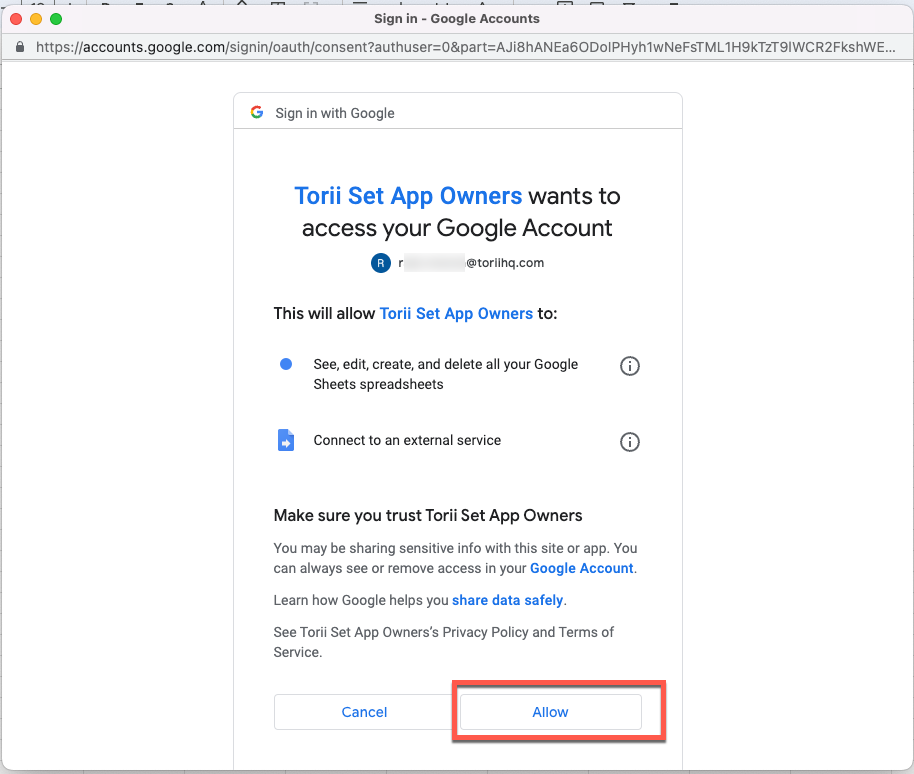
- Enter your API Key when prompted. If you do not have one, please refer to the Getting Started with Torii API guide.
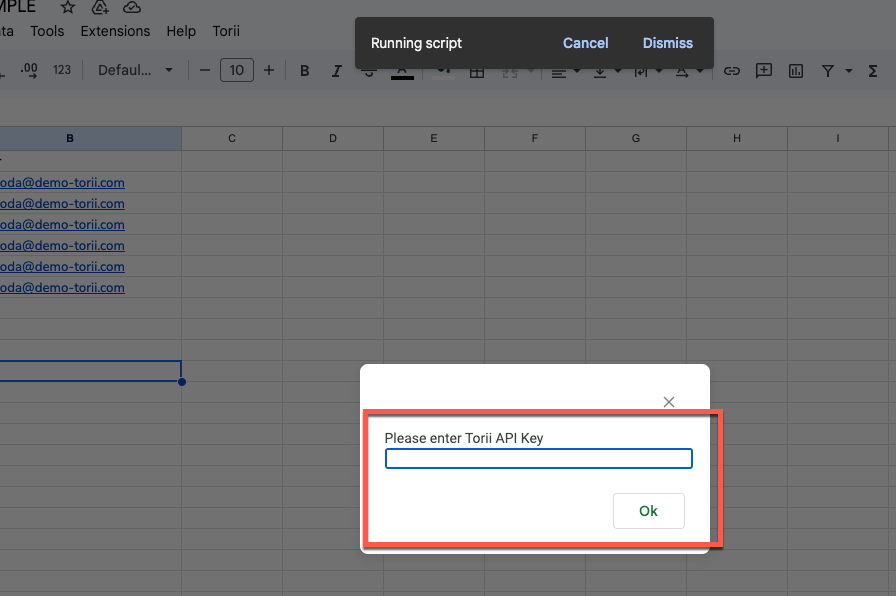
Step 3 - Check the Torii console to verify that the app data has been updated
- Access the Applications tab in the Torii platform, find the applications you've bulk uploaded data for, and verify the app owners match the ones you've listed in your Google Sheet.
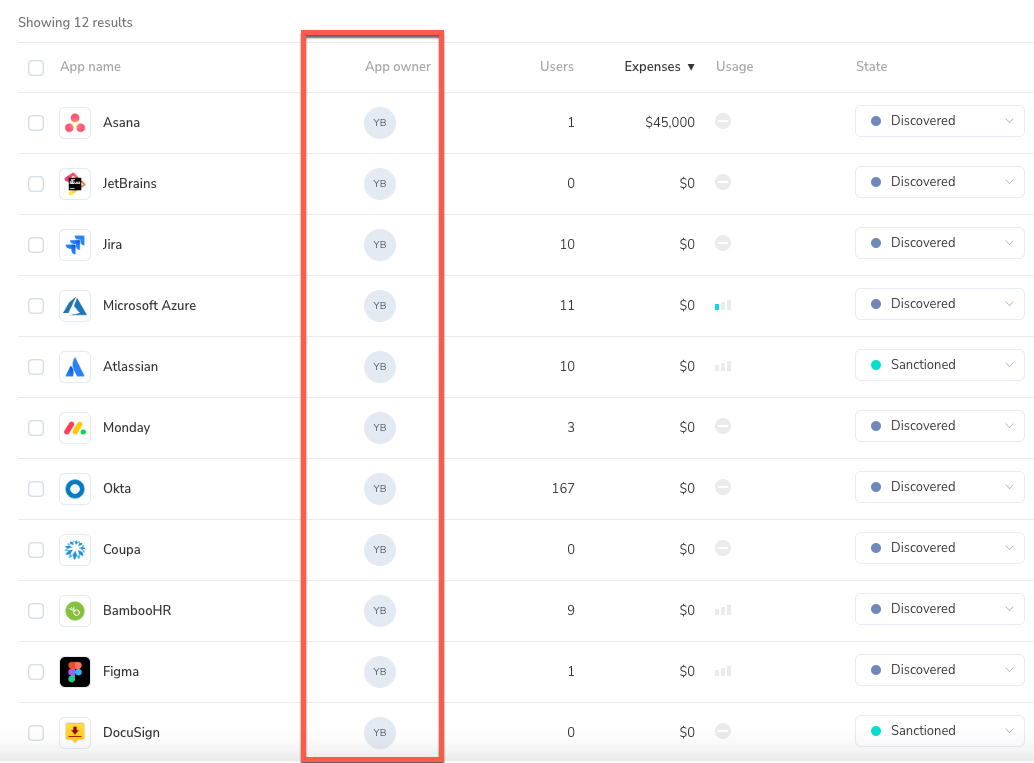
Congratulations! At this point, you should have successfully bulk configured application details in Torii.
Updated about 1 month ago